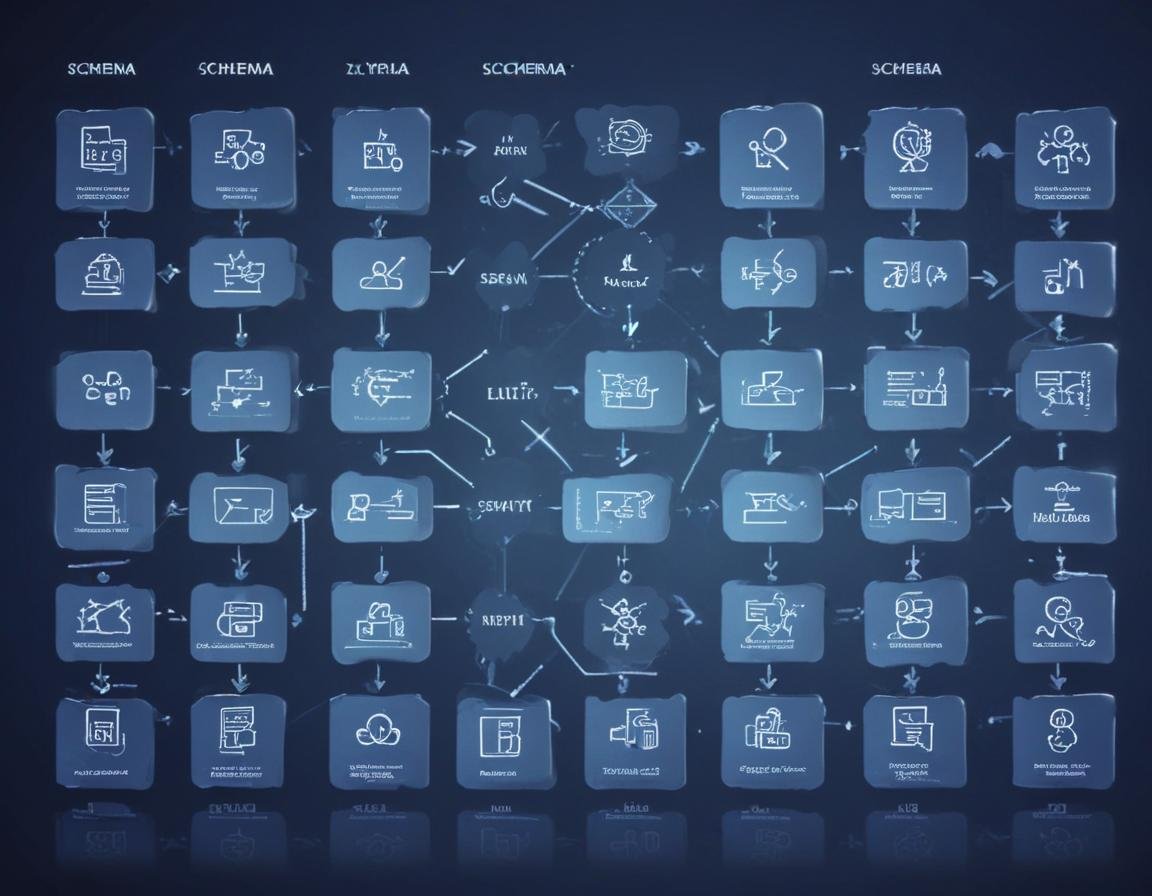
In regular expressions, it’s common to need a match that satisfies multiple conditions simultaneously. This is where lookahead and lookbehind, collectively known as lookaround assertions, come in handy. These zero-width assertions allow the regex engine to test conditions without consuming characters in the string, making it possible to apply multiple requirements to the same portion of text.
Why Lookaround Is Essential
Let’s say you want to match a six-letter word that contains the sequence “cat.” You could achieve this using multiple patterns combined with alternation, like this:
cat\w{3}|\wcat\w{2}|\w{2}cat\w|\w{3}cat
This approach works, but it becomes tedious and inefficient if you need to find words between 6 and 12 letters that contain different sequences like “cat,” “dog,” or “mouse.” In such cases, lookaround simplifies things considerably.
Using Lookahead to Match Multiple Requirements
To break down the process, let’s start with two simple conditions:
The word must be exactly six letters long.
The word must contain the sequence “cat.”
We can easily match a six-letter word using \b\w{6}\b
and a word containing “cat” with \b\w*cat\w*\b
. Combining both requirements with lookahead gives us:
(?=\b\w{6}\b)\b\w*cat\w*\b
Here’s how this works:
The positive lookahead
(?=\b\w{6}\b)
ensures the current position is at the start of a six-letter word.Once the lookahead matches a six-letter word, the regex engine proceeds to check if the word contains “cat.”
If the word contains “cat,” the regex matches the entire word. If not, the engine moves to the next character and tries again.
Optimizing the Regex
While the above solution works, we can optimize it further for better performance. Let’s break down the optimization process:
Removing unnecessary word boundaries
Since the second word boundary\b
is guaranteed to match wherever the first one did, we can remove it:(?=\b\w{6}\b)\w*cat\w*
Optimizing the initial
\w*
In a six-letter word containing “cat,” there can be a maximum of three letters before “cat.” So instead of using\w*
, we can limit it to match up to three characters:(?=\b\w{6}\b)\w{0,3}cat\w*
Adjusting the word boundary
The first word boundary\b
doesn’t need to be inside the lookahead. We can move it outside for a cleaner expression:\b(?=\w{6}\b)\w{0,3}cat\w*
This final regex is more efficient and easier to read. It ensures that the regex engine does minimal backtracking and quickly identifies six-letter words containing "cat."
A More Complex Example
Now, let’s say you want to find any word between 6 and 12 letters long that contains “cat,” “dog,” or “mouse.” You can use a similar approach with a lookahead to enforce the length requirement and a capturing group to match the specific sequences:
\b(?=\w{6,12}\b)\w{0,9}(cat|dog|mouse)\w*
Breaking It Down:
\b(?=\w{6,12}\b)
ensures the word is between 6 and 12 letters long.\w{0,9}
matches up to nine characters before one of the specified sequences.(cat|dog|mouse)
captures the sequence we’re looking for.\w*
matches the remaining characters in the word.
This pattern will successfully match any word within the specified length range that contains one of the target sequences. Additionally, the matching sequence ("cat," "dog," or "mouse") will be captured in a backreference for further use if needed.
Lookaround assertions are powerful tools for creating efficient regular expressions that test multiple conditions on the same portion of text. By understanding how lookahead and lookbehind work and applying optimization techniques, you can create regex patterns that are both effective and efficient. Once you master lookaround, you'll find it invaluable for solving complex text-matching problems in a clean and concise way.
Optimized Example:
\b(?=\w{6}\b)\w{0,3}cat\w*
More Complex Example:
\b(?=\w{6,12}\b)\w{0,9}(cat|dog|mouse)\w*
With these patterns, you can handle even the most complex matching requirements with ease!
Recommended Comments