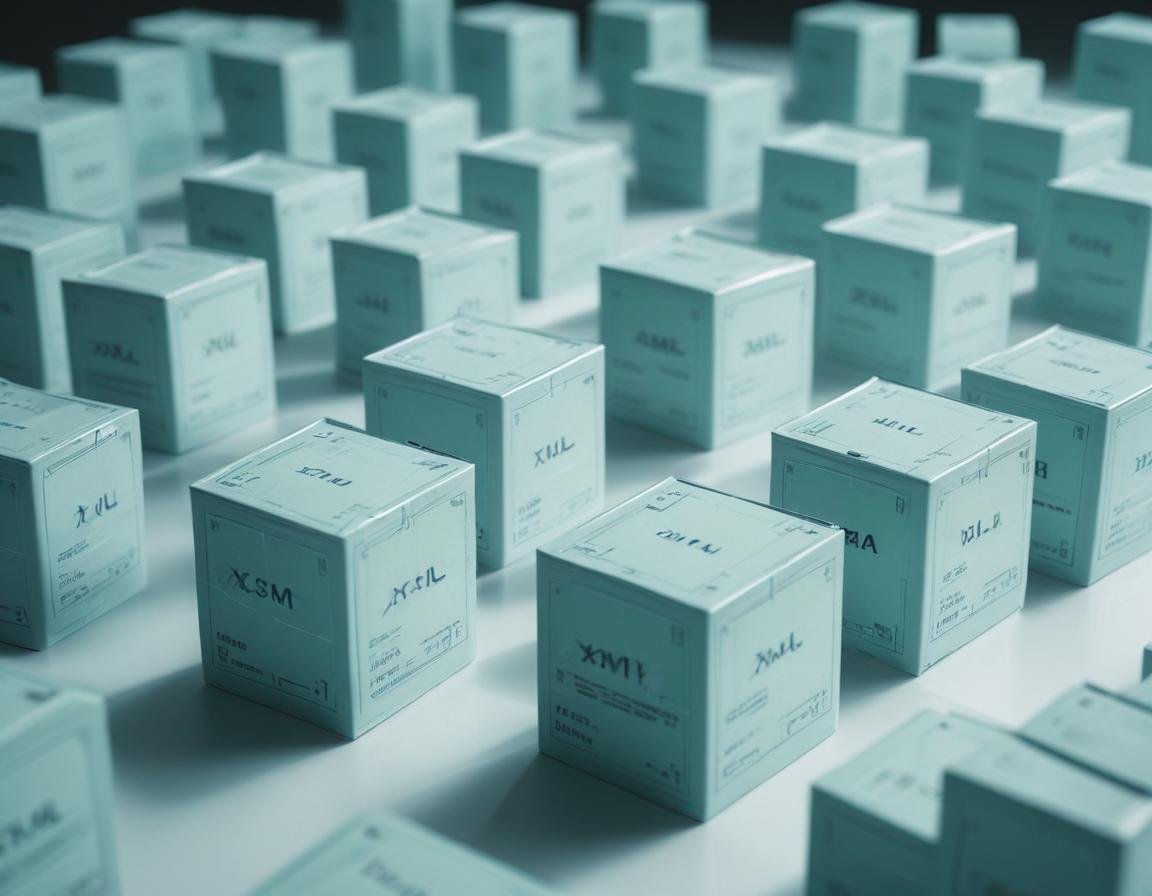
The \G
anchor is a powerful tool in regular expressions, allowing matches to continue from the point where the previous match ended. It behaves similarly to the start-of-string anchor \A
on the first match attempt, but its real utility shines when used in consecutive matches within the same string.
How the \G
Anchor Works
The anchor \G
matches the position immediately following the last successful match. During the initial match attempt, it behaves like \A
, matching the start of the string. On subsequent attempts, it only matches at the point where the previous match ended.
For example, applying the regex \G\w
to the string "test string" works as follows:
The first match finds "t" at the beginning of the string.
The second match finds "e" immediately after the first match.
The third match finds "s", and the fourth match finds the second "t".
The fifth attempt fails because the position after the second "t" is followed by a space, which is not a word character.
This behavior makes \G
particularly useful for iterating through a string and applying patterns step-by-step.
Key Difference: End of Previous Match vs. Start of Match Attempt
The behavior of \G
can vary between different regex engines and tools.
In some environments, such as EditPad Pro,
\G
matches at the start of the match attempt rather than at the end of the previous match.In EditPad Pro, the text cursor’s position determines where
\G
matches. After a match is found, the text cursor moves to the end of that match. As long as you don’t move the cursor between searches,\G
behaves as expected and matches where the previous match left off. This behavior is logical in the context of text editors.
Using \G
in Perl
In Perl, \G
has a unique behavior due to its “magical” position tracking. The position of the last match is stored separately for each string variable, allowing one regex to pick up exactly where another left off.
This position tracking isn’t tied to any specific regex but is instead associated with the string itself. This flexibility allows developers to chain multiple regex patterns together to process a string in a step-by-step manner.
Important Tip: Using the /c
Modifier
If a match attempt fails in Perl, the position tracked by \G
resets to the start of the string. To prevent this, you can use the /c
modifier, which keeps the position unchanged after a failed match.
Example: Parsing an HTML File with \G
in Perl
Here’s a practical example of using \G
in Perl to process an HTML file:
while ($string =~ m/</g) {
if ($string =~ m/\GB>/c) {
# Bold tag
} elsif ($string =~ m/\GI>/c) {
# Italics tag
} else {
# Other tags
}
}
In this example, the initial regex inside the while
loop finds the opening angle bracket (<
). The subsequent regex patterns, using \G
, check whether the tag is a bold (<B>
) or italics (<I>
) tag. This approach allows you to process the tags in the order they appear without needing a massive, complex regex to handle all possible tags at once.
\G
in Other Programming Languages
While Perl offers extensive flexibility with \G
, its behavior in other languages can be more restricted.
In Java, for example, the position tracked by
\G
is managed by theMatcher
object, which is tied to a specific regular expression and subject string. You can manually configure a secondMatcher
to start at the end of the first match, allowing\G
to match at that position.Other languages and engines that support
\G
include .NET, Java, PCRE, and the JGsoft engine.
Summary
The \G
anchor is a valuable tool for continuing regex matches from where the last match left off. While its behavior varies across different tools and languages, it provides a powerful way to process strings incrementally.
Here are a few key takeaways:
Feature | Description |
---|---|
| Matches at the position where the previous match ended |
First Match Behavior | Acts like |
Subsequent Matches | Matches immediately after the last successful match |
Usage in Perl | Tracks the end of the previous match for each string variable |
| Prevents the position from resetting to the start after a failed match |
Supported Languages | .NET, Java, PCRE, JGsoft engine, and Perl |
By understanding \G
, you can write more efficient and maintainable regex patterns that process strings in a structured, step-by-step manner.
Recommended Comments