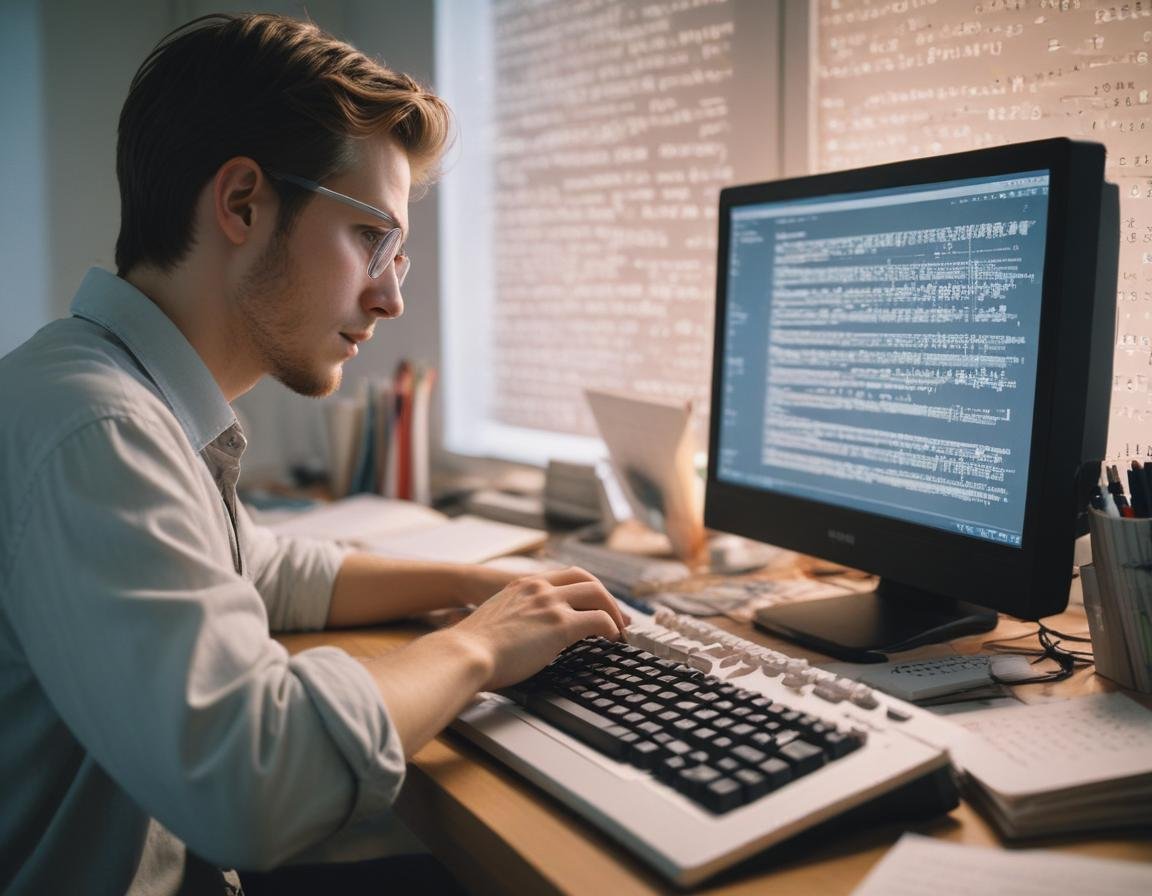
Regular expressions can quickly become complex and difficult to understand, especially when dealing with long patterns. To make them easier to read and maintain, many modern regex engines allow you to add comments directly into your regex patterns. This makes it possible to explain what each part of the expression does, reducing confusion and improving readability.
How to Add Comments in Regular Expressions
The syntax for adding a comment inside a regex is:
(?#comment)
The text inside the parentheses after
?#
is treated as a comment.The regex engine ignores everything inside the comment until it encounters a closing parenthesis
)
.The comment can be anything you want, as long as it does not include a closing parenthesis.
For example, hereβs a regex to match a valid date in the format yyyy-mm-dd, with comments to explain each part:
(?#year)(19|20)\d\d[- /.](?#month)(0[1-9]|1[012])[- /.](?#day)(0[1-9]|[12][0-9]|3[01])
This regex is much more understandable with comments:
(?#year)
: Marks the section that matches the year.(?#month)
: Marks the section that matches the month.(?#day)
: Marks the section that matches the day.
Without these comments, the regex would be difficult to decipher at a glance.
Benefits of Using Comments in Regular Expressions
Adding comments to your regex patterns offers several benefits:
Improves readability: Comments clarify the purpose of each section of your regex, making it easier to understand.
Simplifies maintenance: If you need to update a regex later, comments make it easier to remember what each part of the pattern does.
Helps collaboration: When sharing regex patterns with others, comments make it easier for them to follow your logic.
Using Free-Spacing Mode for Better Formatting
In addition to inline comments, many regex engines also support free-spacing mode, which allows you to add spaces and line breaks to your regex without affecting the match.
Free-spacing mode makes your regex more structured and readable by allowing you to organize it into logical sections. To enable free-spacing mode:
In Perl, PCRE, Python, and Ruby, use the
/x
modifier to activate free-spacing mode.In .NET, use the
RegexOptions.IgnorePatternWhitespace
option.In Java, use the
Pattern.COMMENTS
flag.
Hereβs an example of how free-spacing mode can improve the readability of a regex:
Without Free-Spacing Mode:
(19|20)\d\d[- /.](0[1-9]|1[012])[- /.](0[1-9]|[12][0-9]|3[01])
With Free-Spacing Mode and Comments:
(?#year) (19|20) \d\d # Match years 1900 to 2099
[- /.] # Separator (dash, slash, or dot)
(?#month) (0[1-9] | 1[012]) # Match months 01 to 12
[- /.] # Separator
(?#day) (0[1-9] | [12][0-9] | 3[01]) # Match days 01 to 31
The second version is far easier to read and maintain.
Which Regex Engines Support Comments?
Most modern regex engines support the (?#comment)
syntax for adding comments, including:
Regex Engine | Supports Comments? | Supports Free-Spacing Mode? |
---|---|---|
JGsoft | β Yes | β Yes |
.NET | β Yes | β Yes |
Perl | β Yes | β Yes |
PCRE | β Yes | β Yes |
Python | β Yes | β Yes |
Ruby | β Yes | β Yes |
Java | β No | β
Yes (via |
Example: Using Comments to Document a Complex Regex
Hereβs an example of a more complex regex that extracts email addresses from a text file. Without comments, the regex looks like this:
\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}\b
Adding comments and using free-spacing mode makes it much more understandable:
\b # Word boundary to ensure we're at the start of a word
[A-Za-z0-9._%+-]+ # Local part of the email (before @)
@ # At symbol
[A-Za-z0-9.-]+ # Domain name
\. # Dot before the top-level domain
[A-Za-z]{2,} # Top-level domain (e.g., com, net, org)
\b # Word boundary to ensure we're at the end of a word
Key Points to Remember
Comments in regex are added using the
(?#comment)
syntax.Free-spacing mode makes regex patterns more readable by allowing spaces and line breaks.
Supported engines include JGsoft, .NET, Perl, PCRE, Python, and Ruby.
Java supports free-spacing mode but does not support inline comments.
When to Use Comments and Free-Spacing Mode
Use comments and free-spacing mode when:
Your regex pattern is complex and hard to read.
Youβre working on a team and need to make your patterns understandable to others.
You need to revisit your regex after some time and want to avoid deciphering cryptic patterns.
Adding comments and using free-spacing mode can greatly enhance the readability and maintainability of your regular expressions. Complex patterns become easier to understand, update, and share with others. When working with modern regex engines, take advantage of these features to write cleaner, more maintainable regex patterns.
By making your regex more human-readable, youβll save time and reduce frustration when dealing with intricate text-processing tasks.
Recommended Comments