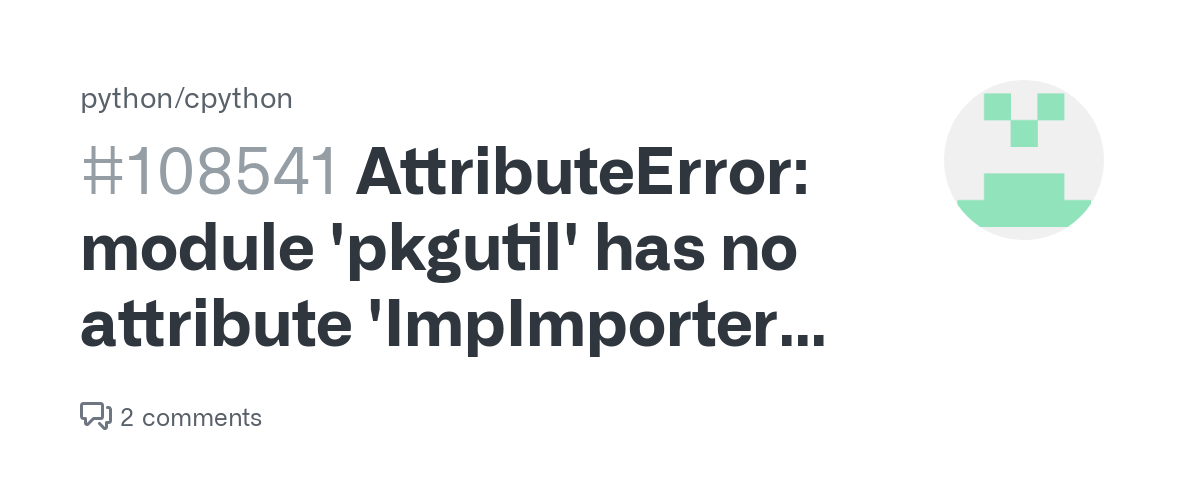
By: Joshua Njiru (cleaned up by ChatGPT)
Thu, 16 Jan 2025 19:44:28 +0000
Understanding the Error
The error "AttributeError: module ‘pkgutil’ has no attribute ‘ImpImporter’" typically occurs in Python code that attempts to use the pkgutil
module to access ImpImporter
. This happens because ImpImporter
was removed in Python 3.12 as part of the deprecation of the old import system.
Root Cause
The removal of ImpImporter
is due to:
The deprecation of the
imp
module in favor ofimportlib
The modernization of Python’s import system
Changes in Python 3.12 that eliminate legacy import mechanisms
Solutions to Fix the Error
Solution 1: Update Your Code to Use importlib
Replace pkgutil.ImpImporter
with the modern importlib
equivalent:
Old Code:
from pkgutil import ImpImporter
New Code:
from importlib import machinery
importer = machinery.FileFinder(path, *machinery.FileFinder.path_hook_for_FileFinder())
Solution 2: Use ZipImporter
Instead
If you’re working with ZIP archives, use ZipImporter
from pkgutil
.
Old Code:
from pkgutil import ImpImporter
New Code:
from pkgutil import ZipImporter
importer = ZipImporter('/path/to/your/zipfile.zip')
Solution 3: Downgrade Python Version
If updating the code isn't possible, downgrade to Python 3.11:
Create a virtual environment with Python 3.11:
python3.11 -m venv env source env/bin/activate # On Unix env\Scripts\activate # On Windows
Install your dependencies:
pip install -r requirements.txt
Code Examples for Common Use Cases
Example 1: Module Discovery
Modern approach using importlib
:
from importlib import util, machinery
def find_module(name, path=None):
spec = util.find_spec(name, path)
if spec is None:
return None
return spec.loader
Example 2: Package Resource Access
Using importlib.resources
:
from importlib import resources
def get_package_data(package, resource):
with resources.path(package, resource) as path:
return path
Prevention Tips
Always check Python version compatibility when using import-related functionality
Use
importlib
instead ofpkgutil
for new codeKeep dependencies updated
Test code against new Python versions before upgrading
Common Pitfalls
Mixed Python versions in different environments
Old dependencies that haven’t been updated
Copying legacy code without checking compatibility
Long-Term Solutions
Migrate to
importlib
completelyUpdate all package loading code to use modern patterns
Implement proper version checking in your application
Checking Your Environment
Run the following diagnostic code to check your setup:
import sys
import importlib
def check_import_system():
print(f"Python version: {sys.version}")
try:
print(f"Importlib version: {importlib.__version__}")
except AttributeError:
print("Importlib does not have a version attribute.")
print("\nAvailable import mechanisms:")
for attr in dir(importlib.machinery):
if attr.endswith('Loader') or attr.endswith('Finder'):
print(f"- {attr}")
if __name__ == "__main__":
check_import_system()
More Articles from Unixmen
The post Fixing "AttributeError: module ‘pkgutil’ has no attribute ‘ImpImporter’" appeared first on Unixmen.
Recommended Comments