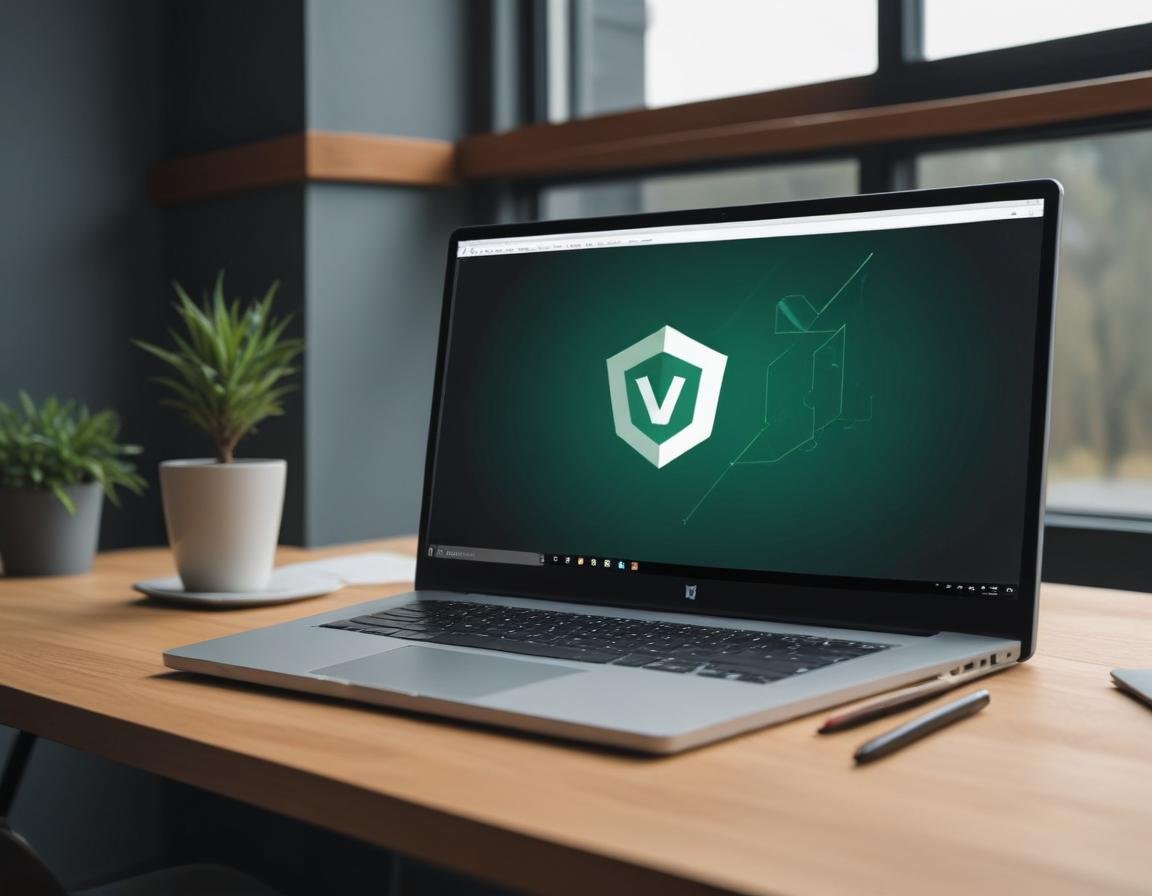
Vue.js is a versatile and progressive JavaScript framework for building user interfaces. Its simplicity and powerful features make it an excellent choice for modern web applications. In this article, we will walk through creating a VueJS application from scratch on both Windows and Linux.
Prerequisites
Before starting, ensure you have the following tools installed on your system:
For Windows:
-
Node.js and npm
- Download and install from Node.js official website.
- During installation, ensure you check the option to add Node.js to your system PATH.
-
Verify installation:
node -v npm -v
-
Command Prompt or PowerShell
- These are pre-installed on Windows and will be used to execute commands.
-
Vue CLI
-
Install globally using npm:
npm install -g @vue/cli
-
Verify Vue CLI installation:
vue --version
-
Install globally using npm:
For Linux:
-
Node.js and npm
-
Install via package manager:
curl -fsSL https://deb.nodesource.com/setup_18.x | sudo -E bash - sudo apt install -y nodejs
-
Replace
18.x
with the desired Node.js version. -
Verify installation:
node -v npm -v
-
Install via package manager:
-
Terminal
- Pre-installed on most Linux distributions and used for executing commands.
-
Vue CLI
-
Install globally using npm:
npm install -g @vue/cli
-
Verify Vue CLI installation:
vue --version
-
Install globally using npm:
-
Curl
- Required for downloading Node.js setup scripts (pre-installed on many distributions, or install via your package manager).
-
Code Editor (Optional)
-
Visual Studio Code (VSCode) is highly recommended for its features and extensions. Install extensions like
Vetur
orVue Language Features
for enhanced development.
-
Visual Studio Code (VSCode) is highly recommended for its features and extensions. Install extensions like
Step-by-Step Guide
1. Setting Up VueJS on Windows
Install Node.js and npm
- Download the Windows installer from the Node.js website and run it.
-
Follow the installation wizard, ensuring
npm
is installed alongside Node.js. -
Verify installation:
node -v npm -v
Install Vue CLI
-
Open a terminal (Command Prompt or PowerShell) and run:
npm install -g @vue/cli vue --version
Create a New Vue Project
-
Navigate to your desired directory:
cd path\to\your\project
-
Create a VueJS app:
vue create my-vue-app
- Choose "default" for a simple setup or manually select features like Babel, Vue Router, or TypeScript.
-
Navigate into the project directory:
cd my-vue-app
-
Start the development server:
npm run serve
-
Open
http://localhost:8080
in your browser to view your app.
2. Setting Up VueJS on Linux
Install Node.js and npm
-
Update your package manager:
sudo apt update sudo apt upgrade
-
Install Node.js:
Replacecurl -fsSL https://deb.nodesource.com/setup_18.x | sudo -E bash - sudo apt install -y nodejs
18.x
with the desired Node.js version. -
Verify installation:
node -v npm -v
Install Vue CLI
-
Install Vue CLI globally:
npm install -g @vue/cli vue --version
Create a New Vue Project
-
Navigate to your working directory:
cd ~/projects
-
Create a VueJS app:
vue create my-vue-app
- Choose the desired features.
-
Navigate into the project directory:
cd my-vue-app
-
Start the development server:
npm run serve
-
Open
http://localhost:8080
in your browser to view your app.
Code Example: Adding a Component
-
Create a new component,
HelloWorld.vue
, in thesrc/components
directory:<template> <div> <h1>Hello, VueJS!</h1> </div> </template> <script> export default { name: "HelloWorld", }; </script> <style scoped> h1 { color: #42b983; } </style>
ย
-
Import and use the component in
src/App.vue
:<template> <div id="app"> <HelloWorld /> </div> </template> <script> import HelloWorld from "./components/HelloWorld.vue"; export default { name: "App", components: { HelloWorld, }, }; </script>
ย
Code Example: MVVM Pattern in VueJS
The Model-View-ViewModel (MVVM) architecture separates the graphical user interface from the business logic and data. Here's an example:
Model
Define a data structure in the Vue component:
export default { data() { return { message: "Welcome to MVVM with VueJS!", counter: 0, }; }, methods: { incrementCounter() { this.counter++; }, }, };
View
Bind the data to the template:
<template> <div> <h1>{{ message }}</h1> <p>Counter: {{ counter }}</p> <button @click="incrementCounter">Increment</button> </div> </template>
ViewModel
The data
and methods
act as the ViewModel, connecting the template (View) with the business logic (Model).
Tips
- Use Vue DevTools for debugging: Available as a browser extension for Chrome and Firefox.
-
Leverage VSCode extensions like
Vetur
orVue Language Features
for enhanced development.
Recommended Comments