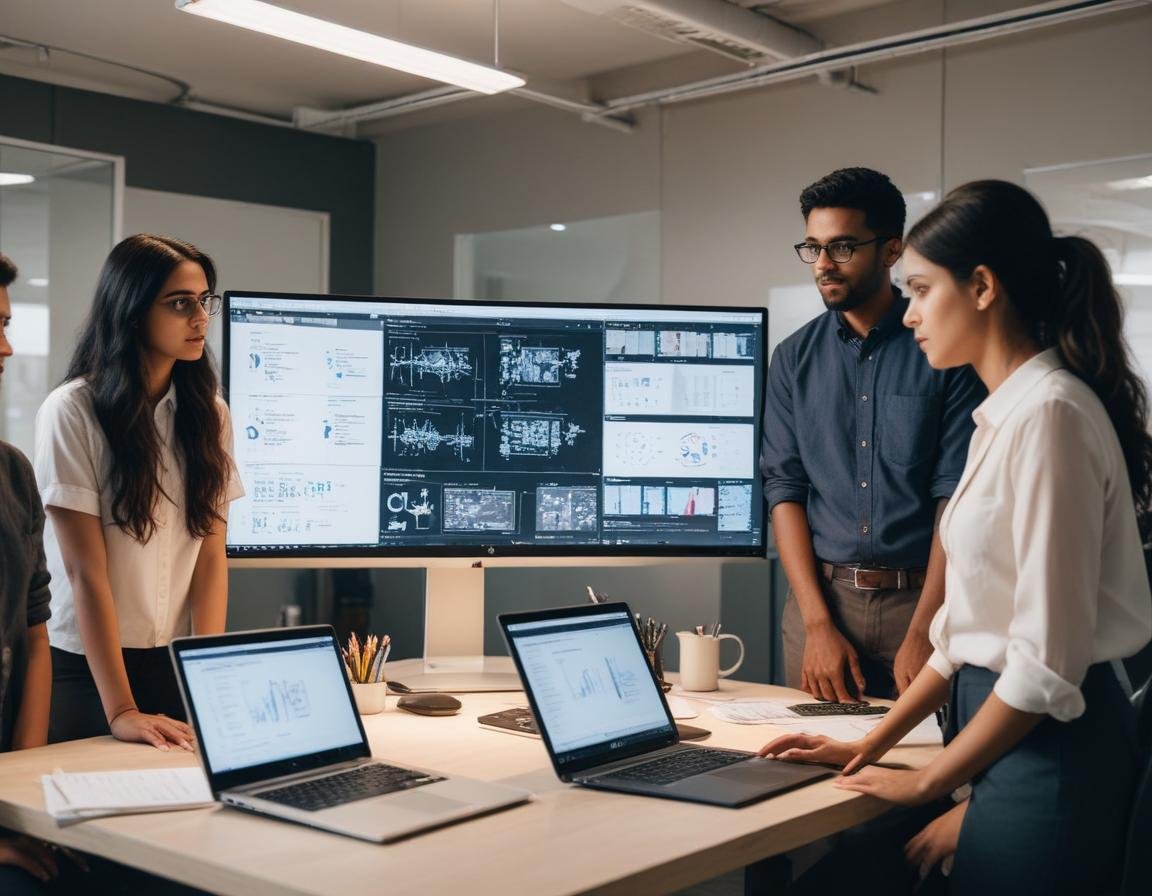
The Model-View-ViewModel (MVVM) architectural pattern is widely used in modern software development for creating applications with a clean separation between user interface (UI) and business logic. Originating from Microsoft's WPF (Windows Presentation Foundation) framework, MVVM has found applications in various programming environments, including web development frameworks like Vue.js, Angular, and React (when combined with state management libraries).
What is MVVM?
The MVVM pattern organizes code into three distinct layers:
1. Model
The Model is responsible for managing the application's data and business logic. It represents real-world entities and operations without any concern for the UI.
-
Responsibilities:
- Fetching, storing, and updating data.
- Encapsulating business rules and validation logic.
-
Examples:
- Database entities, APIs, or data models in memory.
2. View
The View is the visual representation of the data presented to the user. It is responsible for displaying information and capturing user interactions.
-
Responsibilities:
- Rendering the UI.
- Providing elements like buttons, text fields, or charts for user interaction.
-
Examples:
- HTML templates, XAML files, or UI elements in a desktop application.
3. ViewModel
The ViewModel acts as a mediator between the Model and the View. It binds the data from the Model to the UI and translates user actions into commands that the Model can understand.
-
Responsibilities:
- Exposing the Model's data in a format suitable for the View.
- Implementing logic for user interactions.
- Managing state.
-
Examples:
- Observable properties, methods for handling button clicks, or computed values.
Why Use MVVM?
Adopting the MVVM pattern offers several benefits:
-
Separation of Concerns:
- Clear boundaries between UI, data, and logic make the codebase more maintainable and testable.
-
Reusability:
- Components such as the ViewModel can be reused across different views.
-
Testability:
- Business logic and data operations can be tested independently of the UI.
-
Scalability:
- Encourages modularity, making it easier to scale applications as they grow.
MVVM in Practice: Example with Vue.js
Scenario
A simple counter application where users can increment a number by clicking a button.
Implementation
Model
Defines the data and business logic:
export default { data() { return { counter: 0, }; }, methods: { incrementCounter() { this.counter++; }, }, };
View
The template displays the UI:
<template> <div> <h1>Counter: {{ counter }}</h1> <button @click="incrementCounter">Increment</button> </div> </template>
ViewModel
Binds the Model to the View:
export default { name: "CounterApp", data() { return { counter: 0, }; }, methods: { incrementCounter() { this.counter++; }, }, };
Best Practices for Implementing MVVM
-
Keep Layers Independent:
- Avoid tightly coupling the View and Model. The ViewModel should act as the sole intermediary.
-
Leverage Data Binding:
- Utilize frameworks or libraries with robust data binding to keep the View and ViewModel synchronized seamlessly.
-
Minimize ViewModel Complexity:
- Keep the ViewModel focused on presenting data and handling user interactions, not complex business logic.
-
Test Each Layer Separately:
- Write unit tests for the Model and ViewModel and UI tests for the View.
When to Use MVVM?
MVVM is ideal for:
- Applications with complex user interfaces.
- Scenarios requiring significant state management.
- Teams where developers and designers work independently.
Conclusion
The MVVM pattern is a robust architectural solution for creating scalable, maintainable, and testable applications. By clearly separating responsibilities into Model, View, and ViewModel layers, developers can build applications that are easier to develop, debug, and extend. Whether you're working on a desktop application or a modern web application, understanding and implementing MVVM can significantly enhance the quality of your codebase.
Start applying MVVM in your projects today and experience the difference it can make in your development workflow!
Recommended Comments